In this project, TestMenu1.js, we add a Help
menu to the menu bar, and an About item to the Help menu.
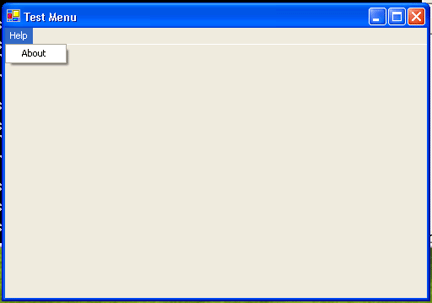
I did find a v1.0 bug when testing this code. If we declare two MenuItem
variables in a single line:
var menuHelp, menuAbout :
System.Windows.Forms.MenuItem;
we get a very unusual menu bar at runtime!
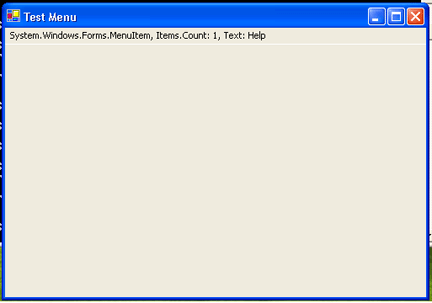
Source Code
import System
import System.Windows.Forms
import System.ComponentModel
import System.Drawing
// this project demonstrates adding a menu bar
package JAL {
class TestMenu extends System.Windows.Forms.Form {
var panelBase : Panel;
var menuMain :
System.Windows.Forms.MainMenu;
// use this syntax
var menuHelp :
System.Windows.Forms.MenuItem;
var menuAbout :
System.Windows.Forms.MenuItem;
// the following syntax results in a
runtime abnormality
// var menuHelp, menuAbout :
System.Windows.Forms.MenuItem;
// constructor
function TestMenu() {
InitializeComponent();
}
// About menu item event handler
private function menuAbout_Clicked(o
: Object, e : EventArgs) {
MessageBox.Show("Test Menu. By JAL.","About");
}
// init form here
private function InitializeComponent()
{
//SuspendLayout()
// name and
center form
Text= "Test
Menu";
ClientSize=
new System.Drawing.Size(500,300);
StartPosition=
System.Windows.Forms.FormStartPosition.CenterScreen;
// create
About menu item
menuAbout =
new System.Windows.Forms.MenuItem();
menuAbout.add_Click(menuAbout_Clicked);
menuAbout.Text = "&About";
menuAbout.ShowShortcut = false;
// create
Help menu and add About menu item
menuHelp =
new System.Windows.Forms.MenuItem();
menuHelp.MenuItems.Add(menuAbout);
menuHelp.Text
= "&Help";
menuHelp.ShowShortcut = false;
// create
Menu Bar
menuMain =
new System.Windows.Forms.MainMenu();
// add Help
menu to menu bar
menuMain.MenuItems.Add(menuHelp);
this.Menu =
menuMain;
// create
base panel
panelBase=
new Panel;
panelBase.Location= new Point(0,0);
panelBase.Size= new System.Drawing.Size(500,300);
panelBase.Name= "panelBase";
// resize in
all directions
panelBase.Anchor= AnchorStyles.Top | AnchorStyles.Left | AnchorStyles.Right |
AnchorStyles.Bottom;
// add base
panel to form
this.Controls.Add(panelBase);
//ResumeLayout()
} // end_initializecomponent
} // end_class
} // end_package
Application.Run(new JAL.TestMenu());
Learn More About Shortcuts
We can easily add a shortcut to the menu item. If the user hits the "A" key while holding
down the "Ctrl" key, the About box will be displayed.
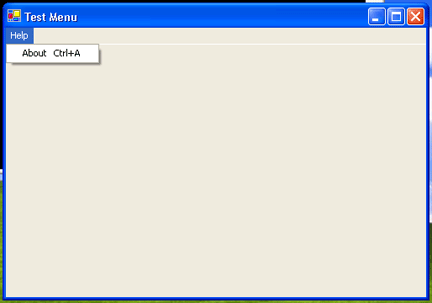
Here is the modified code that adds a shortcut to our project:
// create About menu item
menuAbout = new
System.Windows.Forms.MenuItem();
menuAbout.add_Click(menuAbout_Clicked);
menuAbout.Text = "&About";
menuAbout.ShowShortcut = true;
menuAbout.Shortcut= "CtrlA";
Thats All Folks.
Have fun,
Jeff
Previous FAQ