Palm OS Programming on Windows XP
Get the Toolset
You can download the powerful Eclipse IDE ready for Palm OS development
from
PalmSource at
http://www.palmos.com/dev/dl/dl_tools/dl_pods/index.html
The download includes the Palm SDK and the Palm OS Simulator for testing your
code.
The Eclipse IDE includes a GUI editor, the Palm OS Resource Editor,
that
makes it easy to create forms and alerts.
Sample Code
A trivial encryption program that uses the low security XOR algorithm.
Here is the view of the Palm OS Resource Editor for this project.
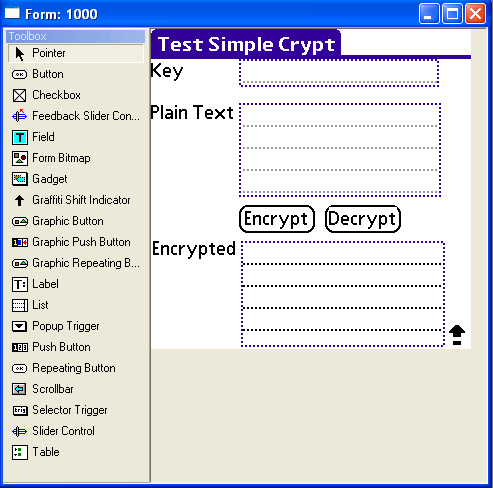
Header File/****************************************************************** TestPalm.h
TestPalm Created by Jeff Louie on Sat Oct 30 2004.
Copyright (c) 2004 __MyCompanyName__. All rights reserved.
*******************************************************************/
// Resource: tFRM 1000
#define MainForm 1000 // Resource: tFRM 1100
#define AboutForm 1100
#define AboutOKButton 1105
#define AboutTitleLabel 1102
#define AboutText1Label 1103
#define AboutText2Label 1104 // Resource: Talt 1001
#define RomIncompatibleAlert 1001
#define RomIncompatibleOK 0
// Resource: MBAR 1000
#define MainFormMenuBar 1000
// Resource: MENU 1000
#define MainOptionsMenu 1000
#define MainOptionsAboutStarterApp 1000
// Resource: PICT 1001
#define Bitmap 1001 // Resource: PICT 1002
#define Bitmap2 1002 // Resource: PICT 1008
#define Bitmap3 1008 // Resource: PICT 1011
#define Bitmap4 1011 // Resource: PICT 1012
#define Bitmap5 1012 // Resource: PICT 1018
#define Bitmap6 1018
// Resource: taif 1000
#define Largeicons12and8bitsAppIconFamily 1000 // Resource: taif 1001
#define Smallicons12and8bitsAppIconFamily 1001
#define DEBUG 1
#define MAX_STRING_LENGTH 255
#define fldKey 1000
#define fldPlain 1002
#define btnEncrypt 1003
#define btnDecrypt 1004
#define fldEncrypt 1006
#define altDebug 1000
#define altInvalidLength 1100
#define altOutOfMemory 1200
Code File
/******************************************************************************
*
* Copyright (c) 1999-2004 PalmSource, Inc. All rights reserved.
*
* File: AppMain.c
*
*****************************************************************************/ #include <PalmOS.h> #include "AppResources.h" /****************************************************************** TestPalm.c
TestPalm Created by Jeff Louie on Sat Oct 30 2004.
Copyright (c) 2004 __MyCompanyName__. All rights reserved.
*******************************************************************/
// BEGIN ALERTS static void SignalInvalidKeyLength() {
FrmCustomAlert(altInvalidLength," Key ",NULL,NULL);
} static void SignalInvalidPlainText() {
FrmCustomAlert(altInvalidLength," Plain Text ",NULL,NULL);
} static void SignalInvalidEncryptedText() {
FrmCustomAlert(altInvalidLength," Encryted Text ",NULL,NULL);
} // END ALERTS // BEGIN UTILITY FUNCTIONS static void Debug(char *message) {
FrmCustomAlert(altDebug,message,NULL,NULL);
} #ifdef DEBUG
static void Assert(BOOL b, char* message) {
if (!b) {Debug(message);}
}
#else
static void Assert(BOOL b, char* message) {;}
#endif static void SignalOutOfMemory() {
FrmAlert(altOutOfMemory);
} // GetObjectPointer
// Get a MemPtr from object in Active Form by object ID
// ASSERT valid object ID for Active Form
static MemPtr GetObjectPointer(UInt16 objectID) {
FormType *frmP;
frmP= FrmGetActiveForm();
return (FrmGetObjectPtr(frmP,FrmGetObjectIndex(frmP,objectID)));
} // GetFieldText Gets text (char*) from a text field in Active Form by object ID
// ASSERT object is a FieldType and valid ID for Active Form
// returns read only const char * client should call StrCopy immediately
// field owns this string and text may change
static const char* GetFieldText(const UInt16 fldID) {
FieldType *fldP;
fldP= (FieldType*)GetObjectPointer(fldID);
if (FldGetTextLength(fldP) > 0 )
{
return FldGetTextPtr(fldP);
}
else {return "";}
}
// SetFieldText eg someField.SetText(message)
// Sets the text in field
// ASSERT object is FieldType and valid ID for active form
// ASSERT message != NULL
static void SetFieldText(UInt16 fldID, char* message) {
MemHandle oldTextH;
char *str;
FieldType *fieldP;
MemHandle textH;
fieldP= (FieldType*)GetObjectPointer(fldID);
textH= MemHandleNew(StrLen(message)+1);
if (!textH) {
SignalOutOfMemory();
return;
}
str= MemHandleLock(textH); // lock memory and get pointer
StrCopy(str,message);
MemHandleUnlock(textH);
oldTextH= FldGetTextHandle(fieldP);
FldSetTextHandle(fieldP,textH); // field must release handle on exit
FldDrawField(fieldP);
if (oldTextH) {MemHandleFree(oldTextH);}
} // END UTILITY FUNCTIONS // BEGIN ALGO // note if char == key --> '\0' and array will not display properly as char*
// ASSERT strlen(encrypted) == strlen(plain)
// ASSERT strlen(key)>0
// return length of encrypted string
static int EncryptIt(int *encrypted, const char *plain, const char *key) {
int keyLength= StrLen(key);
int plainLength= StrLen(plain);
int iKey=0;
int i;
Assert((keyLength>0),"Pre-condition violation in EncryptIt");
for (i=0;*plain;i++) {
*encrypted++= *plain++ ^ key[iKey++];
if (iKey==keyLength) {iKey=0;}
}
return i; // i should == plainLength
} // stops at first null char
// use c library first parameter is lho
static void IntToCharArray(char *out, const int *in) {
while(*in) {
*out++= (char)(*in++);
}
*out= '\0';
} // ASSERT strlen(encrypted) == strlen(plain)
// ASSERT strlen(key)>0
// use c library first parameter is lho
static void DecryptIt(char * plain,
const int* encrypted,
const char * key,
int lengthString) {
int keyLength= StrLen(key);
int iKey= 0;
int i;
for (i=0;i<lengthString && i<MAX_STRING_LENGTH;i++) {
*plain++= *encrypted++ ^ key[iKey++];
if (iKey==keyLength) {iKey=0;}
}
*plain= '\0';
return;
}
// END ALGO
/***********************************************************************
*
* Entry Points
*
***********************************************************************/
/***********************************************************************
*
* Internal Constants
*
***********************************************************************/
#define appFileCreator 'STRT'
#define appVersionNum 0x01
#define appPrefID 0x00
#define appPrefVersionNum 0x01
/***********************************************************************
*
* Internal Functions
*
***********************************************************************/
/***********************************************************************
*
* FUNCTION: MainFormDoCommand
*
* DESCRIPTION: This routine performs the menu command specified.
*
* PARAMETERS: command - menu item id
*
* RETURNED: nothing
*
* REVISION HISTORY:
*
*
***********************************************************************/
static Boolean MainFormDoCommand(UInt16 command)
{
Boolean handled = false;
FormType * pForm; switch (command) {
case MainOptionsAboutStarterApp:
pForm = FrmInitForm(AboutForm);
FrmDoDialog(pForm);
FrmDeleteForm(pForm);
handled = true;
break; }
return handled;
}
/***********************************************************************
*
* FUNCTION: MainFormHandleEvent
*
* DESCRIPTION: This routine is the event handler for the
* "MainForm" of this application.
*
* PARAMETERS: pEvent - a pointer to an EventType structure
*
* RETURNED: true if the event has handle and should not be passed
* to a higher level handler.
*
* REVISION HISTORY:
*
*
***********************************************************************/
static Boolean MainFormHandleEvent(EventType* pEvent)
{
Boolean handled = false;
FormType* pForm;
char *messageC= "";
char key[MAX_STRING_LENGTH+1];
char plain[MAX_STRING_LENGTH+1];
char encrypt[MAX_STRING_LENGTH+1];
int lenKey, lenPlain;
static lenEncrypted=0;
// internal storage encrypted text
static int encryptAsInt[MAX_STRING_LENGTH+1]; switch (pEvent->eType) {
case menuEvent:
return MainFormDoCommand(pEvent->data.menu.itemID); case frmOpenEvent:
pForm = FrmGetActiveForm();
FrmDrawForm(pForm);
handled = true;
break;
case ctlSelectEvent:
switch (pEvent->data.ctlSelect.controlID) {
case btnEncrypt:
lenKey= StrLen(GetFieldText(fldKey));
if (lenKey <1 || lenKey > MAX_STRING_LENGTH) {
SignalInvalidKeyLength();
break;
}
lenPlain= StrLen(GetFieldText(fldPlain));
if (lenPlain <1 || lenPlain > MAX_STRING_LENGTH) {
SignalInvalidPlainText();
break;
}
StrCopy(key,GetFieldText(fldKey));
StrCopy(plain,GetFieldText(fldPlain));
lenEncrypted= EncryptIt(encryptAsInt,plain,key);
IntToCharArray(encrypt,encryptAsInt);
SetFieldText(fldEncrypt,encrypt); // may be truncated by null char
SetFieldText(fldPlain,messageC); // clear field
break;
case btnDecrypt:
lenKey= StrLen(GetFieldText(fldKey));
if (lenKey <1 || lenKey > MAX_STRING_LENGTH) {
SignalInvalidKeyLength();
break;
}
StrCopy(key,GetFieldText(fldKey));
DecryptIt(plain, encryptAsInt, key, lenEncrypted);
SetFieldText(fldPlain,plain);
SetFieldText(fldEncrypt,messageC);
break;
default:
break;
}
break;
default:
break;
}
return handled;
}
/***********************************************************************
*
* FUNCTION: AppHandleEvent
*
* DESCRIPTION: This routine loads form resources and set the event
* handler for the form loaded.
*
* PARAMETERS: event - a pointer to an EventType structure
*
* RETURNED: true if the event has handle and should not be passed
* to a higher level handler.
*
* REVISION HISTORY:
*
*
***********************************************************************/
static Boolean AppHandleEvent(EventType* pEvent)
{
UInt16 formId;
FormType* pForm;
Boolean handled = false; if (pEvent->eType == frmLoadEvent) {
// Load the form resource.
formId = pEvent->data.frmLoad.formID;
pForm = FrmInitForm(formId);
FrmSetActiveForm(pForm); // Set the event handler for the form. The handler of the currently
// active form is called by FrmHandleEvent each time is receives an
// event.
switch (formId) {
case MainForm:
FrmSetEventHandler(pForm, MainFormHandleEvent);
break; default:
break;
}
handled = true;
}
return handled;
}
/***********************************************************************
*
* FUNCTION: AppStart
*
* DESCRIPTION: Get the current application's preferences.
*
* PARAMETERS: nothing
*
* RETURNED: Err value errNone if nothing went wrong
*
* REVISION HISTORY:
*
*
***********************************************************************/
static Err AppStart(void)
{
FrmGotoForm(MainForm);
return errNone;
}
/***********************************************************************
*
* FUNCTION: AppStop
*
* DESCRIPTION: Save the current state of the application.
*
* PARAMETERS: nothing
*
* RETURNED: nothing
*
* REVISION HISTORY:
*
*
***********************************************************************/
static void AppStop(void)
{
// Close all the open forms.
FrmCloseAllForms();
}
/***********************************************************************
*
* FUNCTION: AppEventLoop
*
* DESCRIPTION: This routine is the event loop for the application.
*
* PARAMETERS: nothing
*
* RETURNED: nothing
*
* REVISION HISTORY:
*
*
***********************************************************************/
static void AppEventLoop(void)
{
Err error;
EventType event; do {
EvtGetEvent(&event, evtWaitForever); if (SysHandleEvent(&event))
continue;
if (MenuHandleEvent(0, &event, &error))
continue;
if (AppHandleEvent(&event))
continue; FrmDispatchEvent(&event); } while (event.eType != appStopEvent);
}
/***********************************************************************
*
* FUNCTION: PilotMain
*
* DESCRIPTION: This is the main entry point for the application.
*
* PARAMETERS: cmd - word value specifying the launch code.
* cmdPB - pointer to a structure that is associated with the launch code.
* launchFlags - word value providing extra information about the launch.
* RETURNED: Result of launch
*
* REVISION HISTORY:
*
*
***********************************************************************/
UInt32 PilotMain(UInt16 cmd, MemPtr cmdPBP, UInt16 launchFlags)
{
Err error = errNone; switch (cmd) {
case sysAppLaunchCmdNormalLaunch:
if ((error = AppStart()) == 0) {
AppEventLoop();
AppStop();
}
break; default:
break;
}
return error;
}
|